QR Codes
warning
The document is a continuation of the previous document, if you have landed directly on this page then, Please read from page Get started.
What are QR Codes?
A QR code (Quick Response code) is a type of matrix barcode used to store data like website url, wifi passwords, contact information, etc..
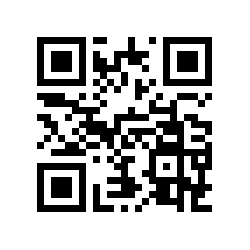
QR code component is used to
- Read QR from input image
- Make QR from input string.
readQR() - Read QR from input image
const char *readQR(cv::Mat Input1, symbol_type_t Input2)
- Description : readQR() takes input as image in OpenCV Mat format & QR code format and returns the QR code data as a (C)string. See Input and Output for details.
- Parameters :
- Input (via Arguments):
- Input1: Image in OpenCV Mat format.
- Input2: QR code format, used to read QR code of type Model 1 and Model 2,
- For Example:
SI_QRCODE
for QR code type Model 1 and Model 2.
- Output :
- on Sucess: Returns QR code data in
const char *
format. - on Failure: Returns Error data in
const char *
format.
- on Sucess: Returns QR code data in
- Input (via Arguments):
makeQR() - Make QR from input string
int8_t makeQR(const char *Input1, const char *Input2)
- Description : makeQR() takes input as Data string and filename and stores the QR code image to disk in PNG format. See Input and Output for details.
- Parameters :
- Input (via Arguments):
- Input1: Data to be encoded as QR in
const char*
format. - Input2: Filename for storing QR generated on disk.
- Input1: Data to be encoded as QR in
- Output :
- on Sucess: Returns 0 in (
int8_t
) format and stores the QR generated as an image in PNG format. - on Failure: Returns -1 in (
int8_t
) format.
- on Sucess: Returns 0 in (
- Input (via Arguments):
List of QR Code features implemented in Shunya stack
With Shunya stack you can make IoT device to act as a
- QR Code Reader.
- QR Code Maker.
note
Shunya Stack currently only supports QR code Type : QR code Model 1 and Model 2
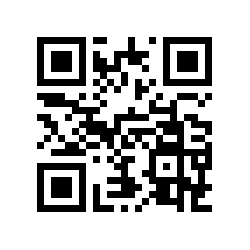
Using QR Code Shunya stack
Requirements to use QR Code Shunya stack
- Shunya OS installed (supported Arm devices) or Shunya OS docker container (X86 based windows/linux devices)
- Camera for Reading QR from live feed. (optional)
Steps to use QR Shunya stack
- Implementation as a QR Code Reader
- Implementation as a QR Code Maker
note
Run the steps given below inside Shunya OS installed (supported Arm devices) or Shunya OS docker container (X86 based windows/linux devices) terminals.
Step 1: Implementation as a QR Code Reader
Let's try to implement QR Code Reader using Shunya stack
Steps are :
Start with an ready to use template,
git clone https://gitlab.iotiot.in/repo-public/examples.git
cd examples/simple-examples/qrcode-reader-imageOpen the
qrcode-reader.cpp
in an text editor and modify as per your use case.For our example, we need to first read the input image, add the code in your
main()
function/* Read image using OpenCV */
cv::Mat image = imread("qrcode.png");To read the QR code in the image, modify the code
/* Find and read QR codes in the image */
std::string qrData = readQR(image, SI_QRCODE);
/* Check if the QR code was read successfully */
if (qrData.compare(0, strlen("ERROR"), "ERROR") == 0) {
std::cout<<"Error: " << qrData <<std::endl;
} else {
std::cout<<"QR Data: " << qrData <<std::endl;
}More examples of QR code Reader in shunya stack
- Read QR code in a video
For reading QR code in a video/camera live feed, change code in step 3 and 4. with the example given below.
captureObj src;
src = newCaptureDevice("video-source"); /* Create capture Instance */
cv::Mat frame; /* Variable to store frame */
while (1) {
frame = captureFrameToMem(&src); /* Capture one frame at a time in a loop*/
if (frame.empty()) {
fprintf(stderr, "End of video file!.");
closeCapture(&src);
return EXIT_SUCCESS;
}
/* Find and read QR codes in the frame */
std::string qrData = readQR(image, SI_QRCODE);
/* Check if the QR code was read successfully */
if (qrData.compare(0, strlen("ERROR"), "ERROR") == 0) {
std::cout<<"Error: " << qrData <<std::endl;
} else {
std::cout<<"QR Data: " << qrData <<std::endl;
}
}
closeCapture(&src);
Step 3: Implementation as a QR Code Maker
Let's try to implement a QR Code Maker using Shunya stack
Steps are :
Start with an ready to use template
git clone https://gitlab.iotiot.in/repo-public/examples.git
cd examples/simple-examples/qrcode-makerOpen the
qrcode-maker.cpp
in an text editor and modify as per your use case.For our example, add this to your
main()
to make qr code which leads to websitehttps://shunyaos.org
./* Generate QR code and store to output_qr.png */
int8_t rc = makeQR("https://shunyaos.org", "output_qr.png");
if (rc < 0) {
std::cerr<<"Error! Failed to generate QR code"<<std::endl;
}The code will make qrcode and store the output into
output_qr.png
file.More examples on making QR code via shunya stack
- Wifi QRcode
For making qrcode which connects to Wifi on read, change code in step 3. with the example given below.
/* Generate QR code and store to output_qr.png */
int8_t rc = makeQR("WIFI:S:<ssid>;T:WPA;P:<password>;;", "output_qr.png");
Understand this component with an example (ready to use code)
Let's take an example use case: Say we need to
- Read QR code from live video feed.
- We will be using video component for capturing live camera feed.
Steps are :
For our example, we need to first set input video to camera,
- Please check here, how to setup the video source path in video component.
Start with an ready to use template for MQTT
git clone https://gitlab.iotiot.in/repo-public/examples.git
cd examples/full-examples/qrcodeOpen the
qrcode-reader.cpp
in an text editor and modify as per your use case.Load the video input settings, add the code in your
main()
functioncaptureObj src;
src = newCaptureDevice("video-source"); /* Argument = JSON Title, Load settings from JSON file */Capture the camera feed frame by frame, add the code in your
main()
functioncv::Mat frame; /* Variable to store frame */
while (1) {
frame = captureFrameToMem(&src); /* Capture one frame at a time in a loop*/
if (frame.empty()) {
fprintf(stderr, "End of video file!.");
closeCapture(&src);
return EXIT_SUCCESS;
}
}Read QRcode in all the frames, add the code in your
while(1)
loop/* Find and read QR codes in the frame */
std::string qrData = readQR(frame);
/* Check if the QR code was read successfully */
if (qrData.compare(0, strlen("ERROR"), "ERROR") == 0) {
std::cout<<"Error: " << qrData <<std::endl;
} else {
std::cout<<"QR Data: " << qrData <<std::endl;
}At the end close video input,
closeCapture(&src);
Finally your code should look like this,
#include <iostream>
#include <cstdlib>
#include <string>
#include <errno.h>
#include <opencv2/opencv.hpp>
#include "si/video.h"
#include "si/scanner.h"
using namespace std;
using namespace cv;
using namespace zbar;
int main(int argc, char *argv[])
{
captureObj src;
src = newCaptureDevice("video-source"); /* Create capture Instance */
Mat frame; /* Variable to store frame */
while (1) {
frame = captureFrameToMem(&src); /* Capture one frame at a time in a loop*/
if (frame.empty()) {
fprintf(stderr, "End of video file!.");
closeCapture(&src);
return EXIT_SUCCESS;
}
/* Find and read QR codes in the frame */
std::string qrData = readQR(frame);
/* Check if the QR code was read successfully */
if (qrData.compare(0, strlen("ERROR"), "ERROR") == 0) {
std::cout<<"Error: " << qrData <<std::endl;
} else {
std::cout<<"QR Data: " << qrData <<std::endl;
}
}
closeCapture(&src);
return EXIT_SUCCESS;
}Once you are done editing, save and compile the code , by running
mkdir build && cd build
cmake ../
makeRun the code
sudo ./qrcode-reader