Thingsboard
warning
The document is a continuation of the previous document, if you have landed directly on this page then, Please read from page Get started.
What is ThingsBoard ?
ThingsBoard is an open-source IoT platform (server) for data collection, processing, visualization and device management.
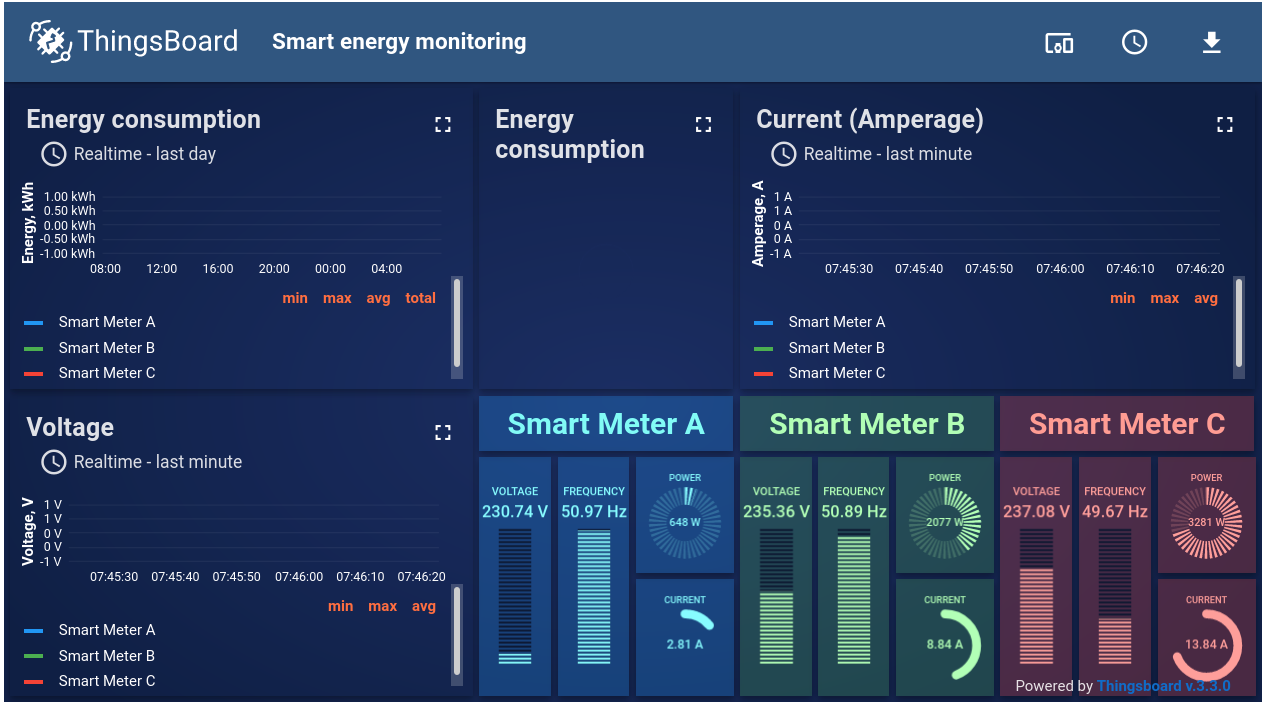
ThingsBoard component is used to send data to the ThingsBoard server.
thingsBoardPublishMqttTm() - Send data to ThingsBoard server
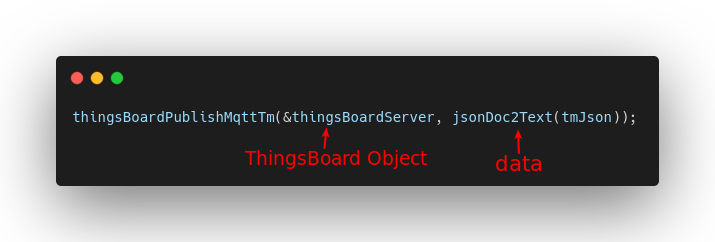
- Description : thingsBoardPublishMqttTm() takes input as thingsboard object & data in JSON string format and returns 0 on Success and -1 on Failure. See Input and Output for details.
- Parameters :
- Input (via Arguments):
- Input1: thingsboard object.
- Input2: data in JSON string format,
- For example:
{ "temperature": 87.9, "humidity": 98.8 }
- For example:
- Output :
- on Sucess: Returns 0.
- on Failure: Returns -1.
- Input (via Arguments):
List of ThingsBoard features implemented in Shunya stack
With Shunya stack you can Send data collected by IoT device to ThingsBoard server.
Using ThingsBoard component
Requirements to use ThingsBoard component
- Shunya OS installed (supported Arm devices) or Shunya OS docker container (X86 based windows/linux devices)
- ThingsBoard server installed and running, easiest way to do this is to use the Thingboard Demo server
- Provision a ThingsBoard Device
Steps to use ThingsBoard component
- Set ThingsBoard server settings in JSON.
- Load JSON settings to a thingsboard object.
- Connect to thingsboard server.
- Get data and convert to JSON.
- Send data to thingsboard.
- Disconnect from server at the end.
note
Run the steps given below inside Shunya OS installed (supported Arm devices) or Shunya OS docker container (X86 based windows/linux devices) terminals.
Step 1: Set ThingsBoard server settings in JSON
Set ThingsBoard server details, by editing the config file /etc/shunya/config.json
inside Shunya OS.
A simple configuration should contain these parameters.
Config | Description |
---|---|
thingsBoardServerUrl | ThingsBoard Server url |
thingsBoardServerPort | ThingsBoard Server Port |
thingsBoardAccessToken | Access Token for ThingsBoard authentication. (optional) |
thingsBoardUsername | Username for ThingsBoard authentication. (optional) |
thingsBoardPassword | Password for ThingsBoard authentication. (optional) |
thingsBoardDeviceId | Unique ID for each device assigned by ThingsBoard. |
note
Thingsboard has 2 types of authentication
- Access Token based
- Username & Password based
Default authentication is Access Token based.
If you are using Access Token then Username & Password are optinal and vice-versa.
Shunya stack will warn you of missing configuration, but will still connect as long as any one type of Thingsboard authentication is provided.
Example configuration:
Writing the below json in /etc/shunya/config.json
file, will tell the Shunya stack to connect to ThingsBoard server at url https://demo.thingsboard.io
"thingsboardSettings": {
"thingsBoardServerUrl": "https://demo.thingsboard.io",
"thingsBoardServerPort": 1883,
"thingsBoardAccessToken": "GVgwJWOSKnjRiaNoKfxK",
"thingsBoardDeviceId": "2ab98b30-9466-11eb-9587-b30e83eb6bf1"
}
You can get all the required settings from Thingsboard UI.
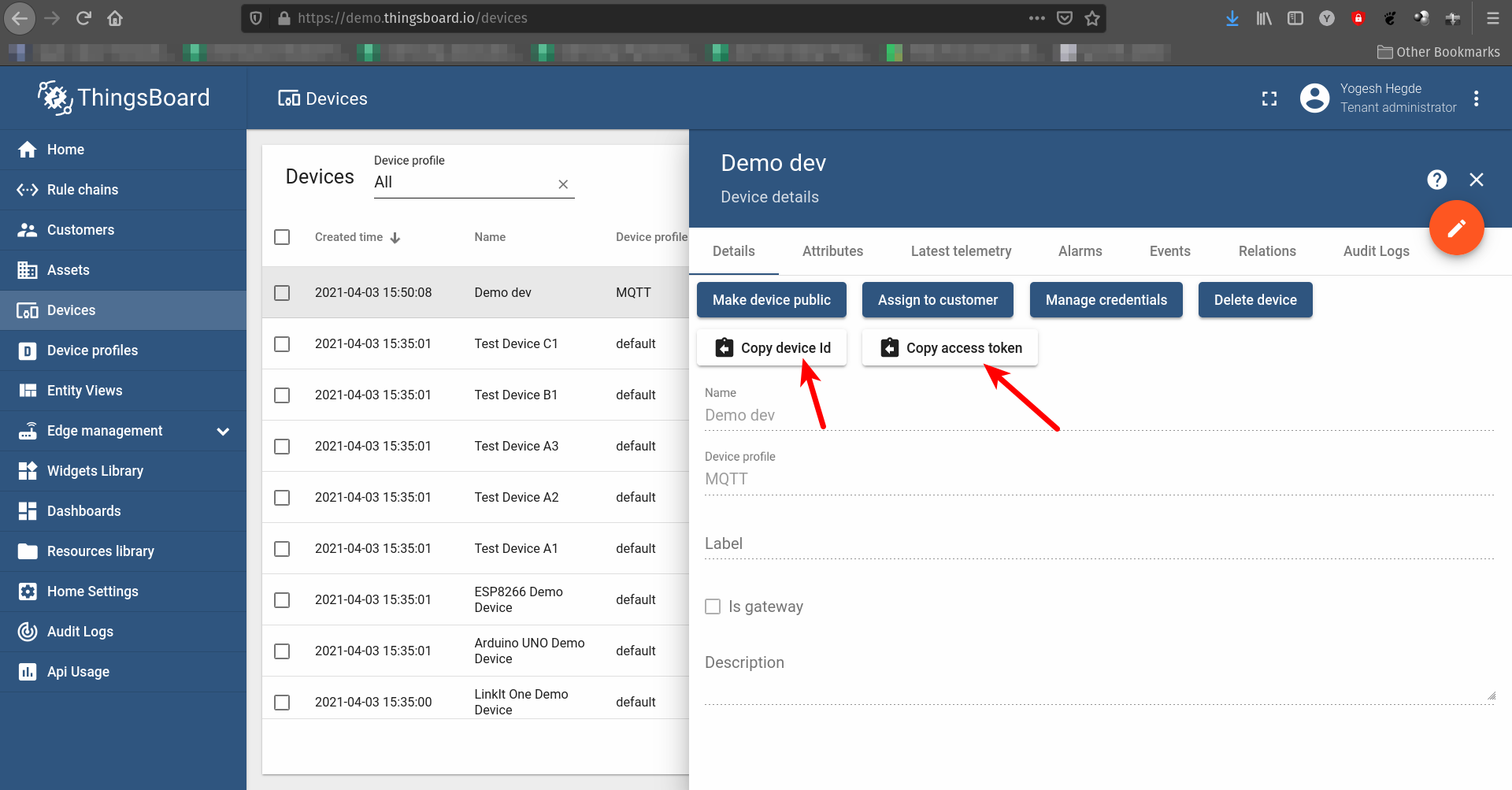
Step 2: Load JSON settings to a thingsboard object.
Start with an ready to use template,
git clone https://gitlab.iotiot.in/repo-public/examples.git
cd examples/simple-examples/thingsboardOpen the
thingsboard.cpp
in an text editor and modify as per your use case.Load JSON settings, add this code to
main()
,/* Load JSON settings to thingsboard object */
thingsBoardObj thingsBoardServer = newThingsBoard("thingsboardSettings"); /* Argument = JSON Title */
Step 3: Connect to thingsboard server.
- To Connect to thingsboard server, add this code to
main()
,/* Connect to thingsboard server */
int rc = thingsBoardConnectMqttTm(&thingsBoardServer);
/* Check if connected Successfully */
if (rc < 0) {
fprintf(stderr, "Error! Unable to connect to Thingsboard server. Error Code %d", rc);
} else {
frpintf(stdout, "Connected to thingsboard server successfully.");
}
Step 4: Get data and convert to JSON.
Use other Shunya components to gather data, for now we will use fixed values.
float temperature = 27.89;
float humidity = 89;To Convert data to JSON format, add this code to
main()
,/* Create JSON for sending data to thingsboard */
rapidjson::Document tmJson;
tmJson.SetObject();
tmJson.AddMember("temperature", temperature, tmJson.GetAllocator());
tmJson.AddMember("humidity", humidity, tmJson.GetAllocator());This will convert your data into JSON given below.
{"temperature": 27.89, "humidity": 89}
Step 5: Send data to thingsboard.
To send data to thingsboard, add this code to
main()
,/* Send data to thingsboard via MQTT */
rc = thingsBoardPublishMqttTm(&thingsBoardServer, jsonDoc2Text(tmJson));
/* Check if connected Successfully */
if (rc < 0) {
fprintf(stderr, "Error! Failed to send data to Thingsboard server. Error Code %d", rc);
} else {
frpintf(stdout, "Data sent to thingsboard server successfully.");
}
Step 6: Disconnect from server at the end.
Finally when all the data is sent disconnect from the thingsboard server, To disconnect, add this code to
main()
,/* Disconnect from the thingboard at the end */
thingsBoardDisconnectMqttTm(&thingsBoardServer);
Understand this component with an example (ready to use code)
Let's take an example use case: Say we need to
- Read Moisture and send the data to Thingsboard.
- We will be using Analog Sensor component to read Moisture sensor.
- And using the Thingsboard component to send data.
Steps are :
Set the Thingsboard server settings in JSON,
Write the below json in
/etc/shunya/config.json
file"thingsboardSettings": {
"thingsBoardServerUrl": "https://demo.thingsboard.io",
"thingsBoardServerPort": 1883,
"thingsBoardAccessToken": "GVgwJWOSKnjRiaNoKfxK",
"thingsBoardDeviceId": "2ab98b30-9466-11eb-9587-b30e83eb6bf1",
}Start with an ready to use template,
git clone https://gitlab.iotiot.in/repo-public/examples.git
cd examples/full-examples/thingsboardOpen the
thingsboard.cpp
in an text editor and modify as per your use case.Load JSON settings,
/* Load JSON settings to thingsboard object */
thingsBoardObj thingsBoardServer = newThingsBoard("thingsboardSettings"); /* Argument = JSON Title */Connect to thingsboard server,
/* Connect to thingsboard server */
int rc = thingsBoardConnectMqttTm(&thingsBoardServer);
/* Check if connected Successfully */
if (rc < 0) {
fprintf(stderr, "Error! Unable to connect to Thingsboard server. Error Code %d", rc);
} else {
frpintf(stdout, "Connected to thingsboard server successfully.");
}Read moisture data from ADC.
/* Read Moisture from sensor */
int16_t moisture1 = getADC(1);
int16_t moisture2 = getADC(2);
int16_t moisture3 = getADC(3);Convert data to JSON formats,
/* Create JSON for sending data to thingsboard */
rapidjson::Document tmJson;
tmJson.SetObject();
tmJson.AddMember("MoistureSensor1", moisture1, tmJson.GetAllocator());
tmJson.AddMember("MoistureSensor2", moisture2, tmJson.GetAllocator());
tmJson.AddMember("MoistureSensor3", moisture3, tmJson.GetAllocator());Send data to thingsboard,
/* Send data to thingsboard via MQTT */
rc = thingsBoardPublishMqttTm(&thingsBoardServer, jsonDoc2Text(tmJson));
/* Check if connected Successfully */
if (rc < 0) {
fprintf(stderr, "Error! Failed to send data to Thingsboard server. Error Code %d", rc);
} else {
frpintf(stdout, "Data sent to thingsboard server successfully.");
}Finally when all the data is sent disconnect from the thingsboard server,
/* Disconnect from the thingboard at the end */
thingsBoardDisconnectMqttTm(&thingsBoardServer);Finally your code should look like this,
#include <si/shunyaInterfaces.h>
#include "si/json_utils.h"
#include <cstdlib>
#include <ctime>
int main (void)
{
/* Initialize the shunya interfaces library */
initLib();
/* Create a thingsboard object */
thingsBoardObj thingsBoardServer = newThingsBoard("thingsboardSettings");
/* Connect to thingsboard server */
int rc = thingsBoardConnectMqttTm(&thingsBoardServer);
/* Check if connected Successfully */
if (rc < 0) {
fprintf(stderr, "Error! Unable to connect to Thingsboard server. Error Code %d", rc);
} else {
frpintf(stdout, "Connected to thingsboard server successfully.");
}
while(1){
/* Read Moisture from sensor */
int16_t moisture1 = getADC(1);
int16_t moisture2 = getADC(2);
int16_t moisture3 = getADC(3);
/* Create JSON for sending data to thingsboard */
rapidjson::Document tmJson;
tmJson.SetObject();
tmJson.AddMember("MoistureSensor1", moisture1, tmJson.GetAllocator());
tmJson.AddMember("MoistureSensor2", moisture2, tmJson.GetAllocator());
tmJson.AddMember("MoistureSensor3", moisture3, tmJson.GetAllocator());
/* Send data to thingsboard via MQTT */
rc = thingsBoardPublishMqttTm(&thingsBoardServer, jsonDoc2Text(tmJson));
/* Check if connected Successfully */
if (rc < 0) {
fprintf(stderr, "Error! Failed to send data to Thingsboard server. Error Code %d", rc);
} else {
frpintf(stdout, "Data sent to thingsboard server successfully.");
}
/* Set delay to 1 sec, so data is sent every sec */
delay(1000);
}
/* Disconnect from the thingboard at the end */
thingsBoardDisconnectMqttTm(&thingsBoardServer);
return 0;
}Once you are done editing, save and compile the code , by running
mkdir build && cd build
cmake ../
makeRun the code
sudo ./thingsboard